Learn how to set up and use the PHP SDK.
This step-by-step guide leads you through setting up and making API calls using the PHP SDK.
Requirements
To follow this guide you should have the following:
- A commercetools Composable Commerce Project
- An API Client
- PHP 7.2 (or later)
- Composer
Objectives of the get started guide
After following this guide you will have:
Placeholder values
Example code in this guide uses the following placeholder values. You should replace these placeholders with the following values.
Placeholder | Replace with | From |
---|---|---|
{projectKey} | project_key | your API Client |
{clientID} | client_id | your API Client |
{clientSecret} | secret | your API Client |
{scope} | scope | your API Client |
{region} | your Region | Hosts |
Install the PHP SDK
Use the following command to install the PHP SDK:
composer require commercetools/commercetools-sdk
Create the Client class
Client.php
and insert the following code:<?php
namespace Commercetools;
// Required imports
use Commercetools\Api\Client\ApiRequestBuilder;
use Commercetools\Api\Client\ClientCredentialsConfig;
use Commercetools\Api\Client\Config;
use Commercetools\Client\ClientCredentials;
use Commercetools\Client\ClientFactory;
use GuzzleHttp\ClientInterface;
require_once __DIR__ . '/vendor/autoload.php';
class Client
{
function createApiClient()
{
/** @var string $clientID */
/** @var string $clientSecret */
/** @var string $scope
* Provide a specific scope for the client.
* If not included, all the scopes of the OAuth client will be used.
* Format: `{scope name}:{projectKey}`.
* Example: `manage_products:project1`.
*/
$authConfig = new ClientCredentialsConfig(
new ClientCredentials('{clientID}', '{clientSecret}', '{scope}'),
[],
'https://auth.{region}.commercetools.com/oauth/token'
);
$client = ClientFactory::of()->createGuzzleClient(
new Config([], 'https://api.{region}.commercetools.com'),
$authConfig
);
/** @var ClientInterface $client */
$builder = new ApiRequestBuilder($client);
// Include the Project key with the returned Client
return $builder->withProjectKey('{projectKey}');
}
}
?>
Test the Client
In your PHP program, add the following code:
<?php
namespace Commercetools;
// Include the Client
require_once 'Client.php';
// Create the apiRoot with your Client
$apiRoot = (new Client())->createApiClient();
// Make a get call to the Project
$myProject = $apiRoot->get()->execute();
// Output the Project name
echo $myProject->getName();
?>
$apiRoot
to build requests to the Composable Commerce API.$myProject
and outputs the Project's name using getName()
.Use the PHP SDK
Imports
Without importing resource-specific classes you cannot use/access specific objects and methods.
For example, to create a Shopping List you must import:
use Commercetools\Api\Models\ShoppingList\ShoppingListDraftBuilder;
require_once __DIR__ . '/vendor/autoload.php';
If not imported, the SDK returns a "Class not found" fatal error with the name of the required class.
Use builders
The PHP SDK follows a builder pattern when constructing drafts, update actions, and other objects/types that contain multiple fields.
// Imports
use Commercetools\Api\Models\Common\LocalizedStringBuilder;
use Commercetools\Api\Models\Common\MoneyBuilder;
use Commercetools\Api\Models\Category\CategoryDraftBuilder;
require_once __DIR__ . '/vendor/autoload.php';
// Create a LocalizedString
$localizedString = LocalizedStringBuilder::of()
->put('en', 'English value')
->put('de', 'German value')
->build();
// Create US$100.00
$money = MoneyBuilder::of()
->withCurrencyCode('USD')
->withCentAmount(10000)
->build();
// Create a Category
$categoryDraft = CategoryDraftBuilder::of()
->withName(
LocalizedStringBuilder::of()
->put('en', 'English name')
->build()
)
->withSlug(
LocalizedStringBuilder::of()
->put('en', 'english-slug')
->build()
)
->withKey('category-key')
->build();
build()
finishes building the object.Structure your API call
Add an endpoint
$apiRoot
. The following targets the Shopping Lists endpoint:$shoppingListInfo = $apiRoot->shoppingLists();
// ...
If your IDE supports auto-complete, you can see the full list of endpoints.
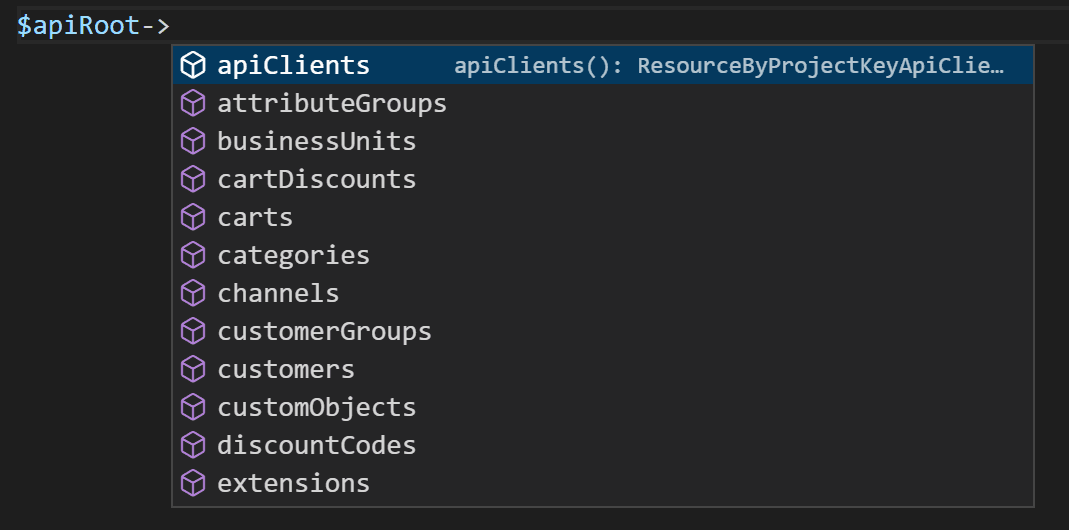
Retrieve data
Get a single resource
get()
and execute()
.// Get a specific Shopping List by ID
$shoppingListInfo = $apiRoot
->shoppingLists()
->withId('a-shoppinglist-id')
->get()
->execute();
// Get a specific Shopping List by key
$shoppingListInfo = $apiRoot
->shoppingLists()
->withKey('a-shoppinglist-key')
->get()
->execute();
$shoppingListInfo
now contains the data of the specified Shopping List. You can access information from the fields within that object: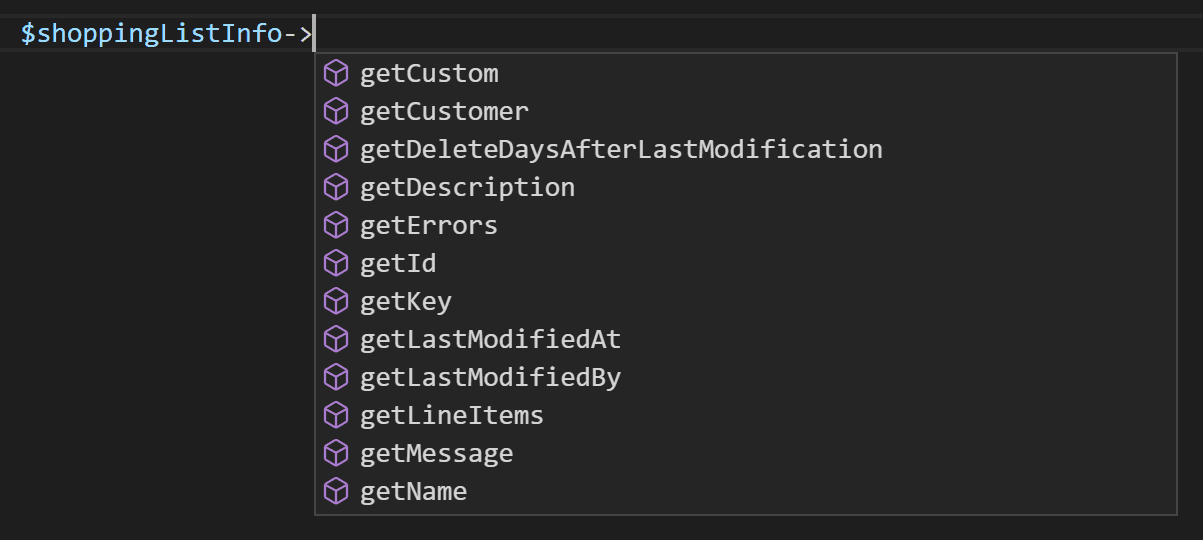
Get multiple resources
PagedQueryResponse
, which is identical to the PagedQueryResults in the HTTP API.// Return a ShoppingListPagedQueryResponse
$shoppingListQuery = $apiRoot
->shoppingLists()
->get()
->execute();
withWhere()
, withSort()
, withExpand()
, withLimit()
, or withOffset()
after get()
.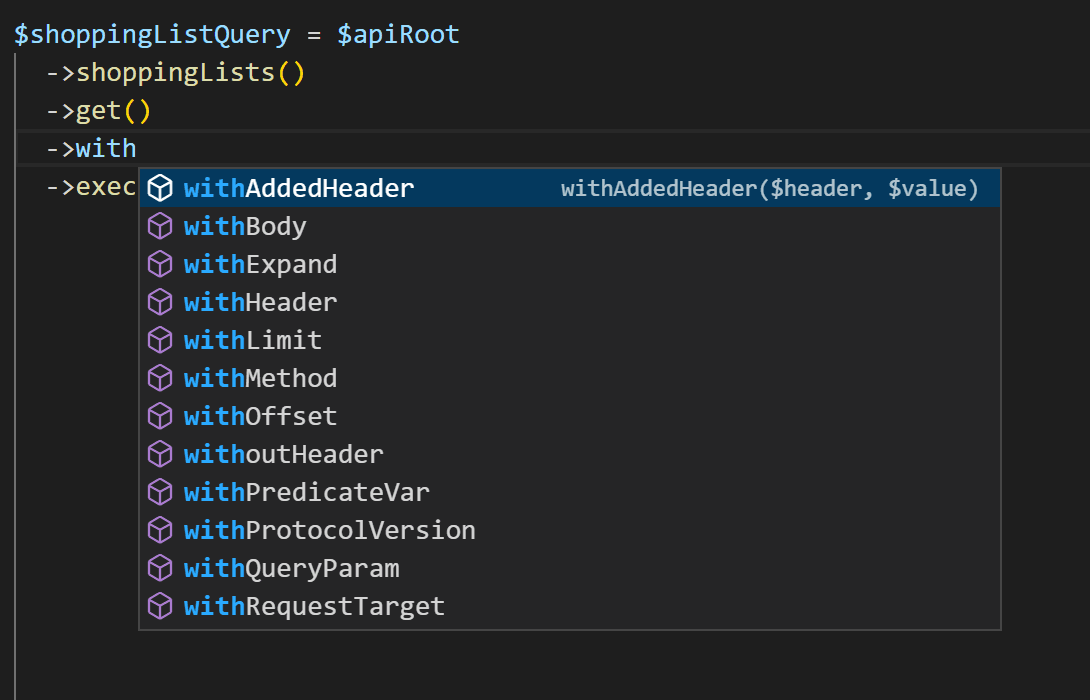
View results
PagedQueryResponse
using getResults()
:// Return a ShoppingListPagedQueryResponse
$shoppingListQuery = $apiRoot
->shoppingLists()
->get()
->execute();
// Put the returned Shopping Lists in a collection
$collectionOfShoppingLists = $shoppingListQuery->getResults();
// Output the first Shopping List's English name
echo $collectionOfShoppingLists[0]->getName()['en'];
Write a resource
Create a new resource
// Required import
use Commercetools\Api\Models\ShoppingList\ShoppingListDraftBuilder;
require_once __DIR__ . '/vendor/autoload.php';
// Build a ShoppingListDraft with the required fields (email address and password)
$newShoppingListDetails = (new ShoppingListDraftBuilder())
->withName(
(new LocalizedStringBuilder())
->put('en', 'English name of Shopping List')
->build()
)
->build();
$newShoppingListDetails
within post()
and follow with execute()
.// Post the ShoppingListDraft and get the new Shopping List
$newShoppingList = $apiRoot
->shoppingLists()
->post($newShoppingListDetails)
->execute();
Update an existing resource
ShoppingListUpdate
) contains a collection of update actions and the last seen version of the resource.// Required imports
use Commercetools\Api\Models\ShoppingList\ShoppingListUpdateBuilder;
use Commercetools\Api\Models\ShoppingList\ShoppingListUpdateActionCollection;
use Commercetools\Api\Models\ShoppingList\ShoppingListSetKeyActionBuilder;
require_once __DIR__ . '/vendor/autoload.php';
// Build a ShoppingListUpdate with the required fields (version and ShoppingListUpdateActionCollection)
$shoppingListUpdate = (new ShoppingListUpdateBuilder())
->withVersion(1)
->withActions(
(new ShoppingListUpdateActionCollection())->add(
(new ShoppingListSetKeyActionBuilder())
->withKey('a-unique-shoppinglist-key')
->build()
)
)
->build();
withId()
or withKey()
).// Post the ShoppingListUpdate and return the updated Shopping List
$updatedShoppingList = $apiRoot
->shoppingLists()
->withId('{shoppingListID}')
->post($shoppingListUpdate)
->execute();
Delete a resource
.delete()
method with the last seen version of the resource. You must identify the resource to delete using withId()
or withKey()
.// Delete and return a Shopping List
$deletedShoppingList = $apiRoot
->shoppingLists()
->withId('{shoppingListID}')
->delete()
->withVersion(1)
->withDataErasure('true') // Include to erase related personal data
->execute();
Retrieve the raw API response
execute()
to return the resource as an instance of an object.send()
.// Return a ShoppingListPagedQueryResponse
$shoppingListQuery = $apiRoot
->shoppingLists()
->get()
->send()
->getBody();
// Output the raw API response
echo $shoppingListQuery;